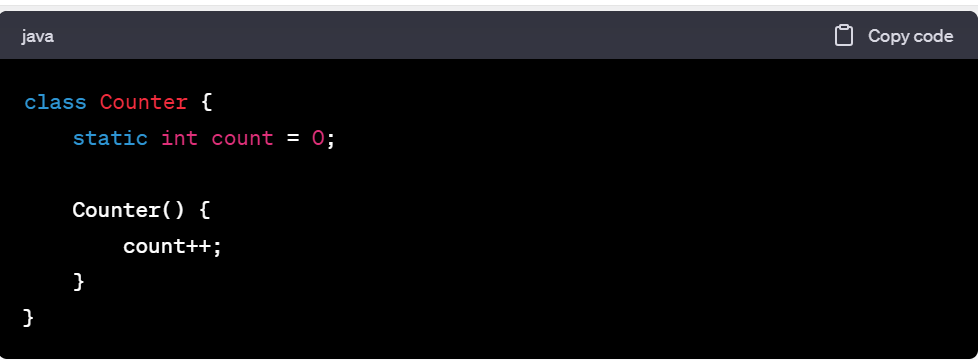
What Does Static Mean in Java: Static in Java Programming
Java, a programming language extensively utilized worldwide, encompasses a multitude of characteristics and principles that enhance its adaptability and potency. Within this array of attributes, the “static” keyword assumes a pivotal role. Comprehending the implications of “static” is imperative for developers aiming to create code that is both efficient and easily maintainable. Within this exposition, we will delve into the realm of this concept and dissect its diverse applications.
The Basics of Static: A Quick Overview
In Java, the term “static” is utilized to declare class-level members that are associated with the class itself as opposed to its occurrences. This implies that the components are accessible to and used by all class instances and can be accessed directly through it, without needing to create an object. These members can include variables, methods, and nested classes.
Static Variables: Sharing Data Across Instances
Static variables, often referred to as class variables, serve as repositories for data intended to be accessible across all occurrences within a class. When a variable is designated in this manner, only one instance of said variable is generated and distributed across every object created from the category. This proves especially advantageous when the objective is to uphold a solitary value across numerous objects belonging to an identical one. For instance, take into account a class denoted as “Counter,” housing a static variable dubbed “count,” which meticulously records the aggregate count of instances that have been instantiated:
In this case, each time a new instance of Counter is created, the count variable will be incremented, reflecting the total number of instances.
Static on Methods
In the realm of Java programming, the reserved term “static” holds a significant role beyond just quantities. It also applies to methods, providing a distinct behavior that can influence how forms are accessed, invoked, and interact with the broader program structure. Let’s explore in more detail the nuances of using this approach on forms and uncover the implications it brings to your coding practices.
Defining Fixed Methods: A Recap
Before delving into the details, let’s review the basics. When a method is declared as so, it becomes linked to the category instead of the samples that compose it. This means that you can call the method using the name directly, without creating an object of it. This unique characteristic has several implications that can affect the design and usage of your Java classes.
1. Method Invocation without Instances
Constant methods are invoked at the group level, which means they are reachable excluding the need to create an instance This is particularly valuable for utility techniques or functions that don’t need access to instance-specific data. You can call this type of method utilizing the type label, followed by the method one.
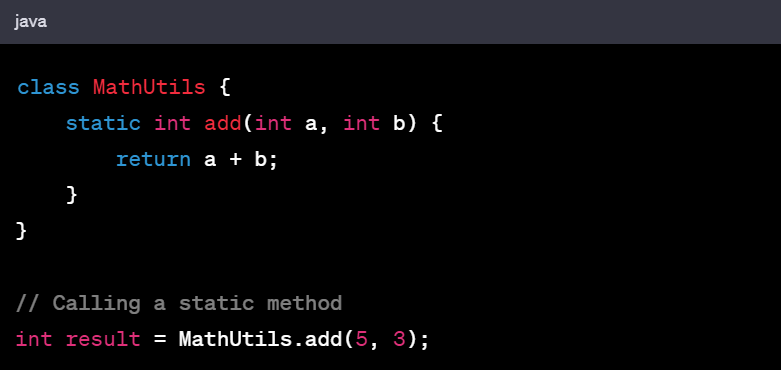
2. Inability to Override Static Methods
In Java, approaches are subject to being replaced in subclasses to provide specialized behavior. Nevertheless, it’s a little bit altered for these procedures. They are resolved at compile-time based on the reference type, not the runtime type. In other words, if a subclass defines a static method with the same signature as the one in the parent class, it won’t override it; instead, it will shadow it.
3. Static Methods and Polymorphism
Due to the inability to override these methods, invoking them using a subclass source still results in the execution of the superclass’s fixed approach. This is because these kinds are resolved at compile-time based on the reference type.
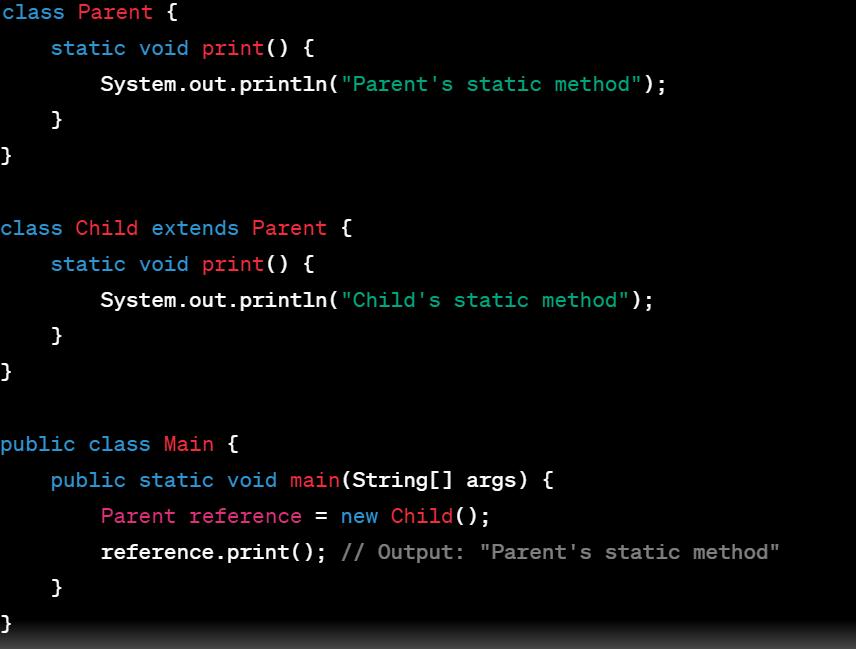
4. Shared State and Thread Safety
They share states across all instances and threads, which can lead to potential thread-safety issues. Care should be taken when accessing or modifying shared fixed variables within static methods. Proper synchronization mechanisms might be necessary to prevent race conditions.
5. Utility Groups and Organization
Those methods are often applied in utility classes to provide common features that don’t need entity instantiation. These categories help organize related operations and promote script reusability.
Static Blocks in Java
When exploring the world of Java programming, the stationary keyword plays a crucial role in static blocks, providing a mechanism for initializing class-level resources and executing code when a class is loaded into memory. Below you can acquire additional details about static blocks in Java, uncovering their purpose, benefits, and best practices.
Understanding Static Blocks: Initialization and Execution
Static blocks, also known as static initializer blocks, are sections of code within a class that are executed only once when it is loaded into memory. These blocks provide a way to perform class-level initialization tasks, such as initializing static variables or setting up resources that need to be available to all cases of the group.
Syntax of Static Blocks
The syntax for a static block is as follows:
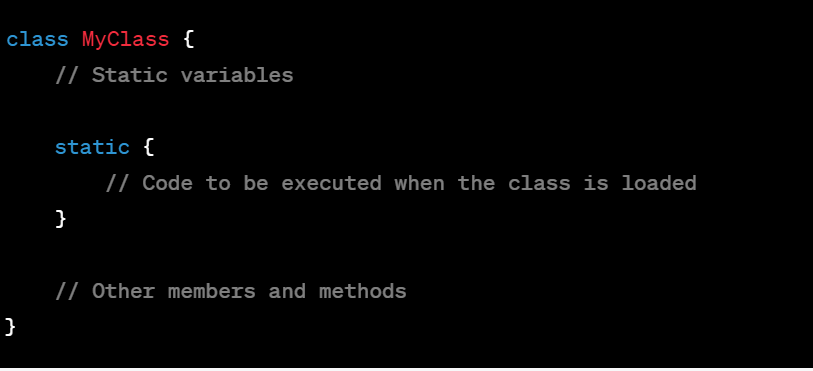
Use Cases for Static Blocks
- Initializing Static Variables: Static blocks are often used to initialize parameters before any element of the class is created. This is useful for cases where the initialization requires complex calculations or loading data from external sources.
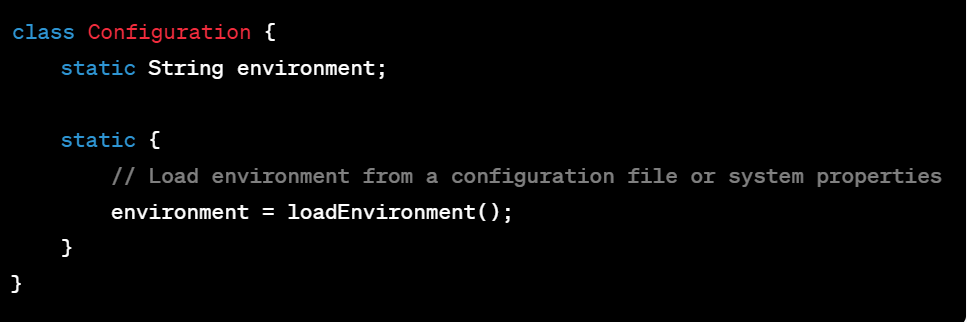
- Loading Native Libraries: If your Java class requires interaction with native libraries or external resources, you can use a static block to load these resources when it is loaded into memory.
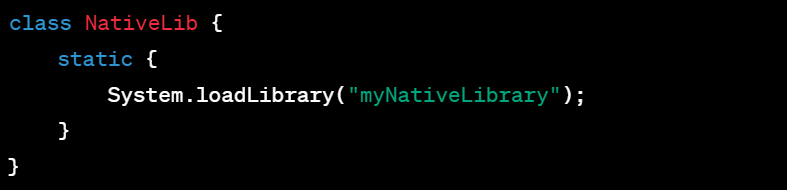
- Setting Up Shared Resources: In scenarios where multiple elements of a category need admission to shared resources, you can use immutable blocks to initialize and manage these resources efficiently.
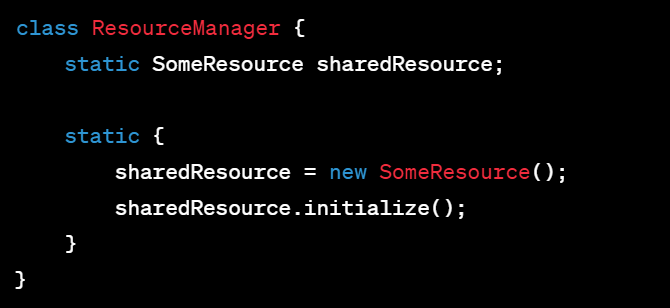
Benefits of Using Static Blocks
- Single Execution. They are executed solely on a single occasion when the class is loaded, ensuring that the initialization code runs precisely when needed, regardless of how many instances are generated;
- Encapsulation. Static blocks allow you to contain complex initialization logic within the category itself, improving code organization and readability;
- Resource Management. They are especially useful for managing resources that are shared among all instances, preventing all leaks or improper initialization.
Limitations and Considerations
- No Control Flow. Static blocks can’t contain control flow statements like loops or conditionals. They are designed for simple initialization tasks;
- Thread Safety. Be cautious when using them to initialize shared resources, as multiple threads might attempt to access the resources simultaneously. Proper synchronization mechanisms might be necessary;
- Exception Handling. Any exceptions thrown within a static block are not caught by the block itself. You need to handle them explicitly, which might involve catching them within the block or letting them propagate to the caller.
Static Nested Classes
A static nested class is a group that is defined within another one, but it is marked with the static keyword. This differentiates it from inner classes, which are non-fixed and have access to the instance parameters and techniques of the outer kind. The nature of these nested groups brings several advantages and use cases to the table.
Defining Static Nested Classes
The syntax for defining a static nested class is as follows:
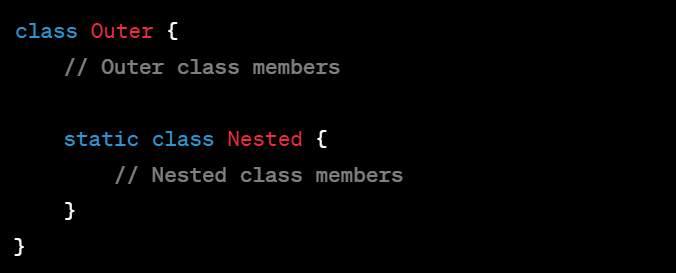
Benefits and Use Cases
- Namespace Arrangement. Static nested categories provide a way to logically group other ones together and create a clear hierarchical structure within your codebase. This improves script arrangement and makes it easier to locate related classes;
- Encapsulation and Privacy. By using this approach, you limit the visibility of the nested class to the outer group and other ones within the same package. This encapsulation ensures that the nested class’s implementation details are hidden from external code;
- Improved Separation of Concerns. It will allow you to define utility classes, helper classes, or small components directly within the categories that use them. This avoids cluttering the package with numerous standalone types;
- Access to Outer Class Members. While static nested classes can’t gain entry to instance elements of the outer category, they can reach stationary elements, including private static members, if they are within the same top-level category.
Example: Using Static Nested Classes for Organization
Consider an application that manages different types of documents. You could use a static nested class to encapsulate the document-related logic and data structures within the main DocumentManager class:
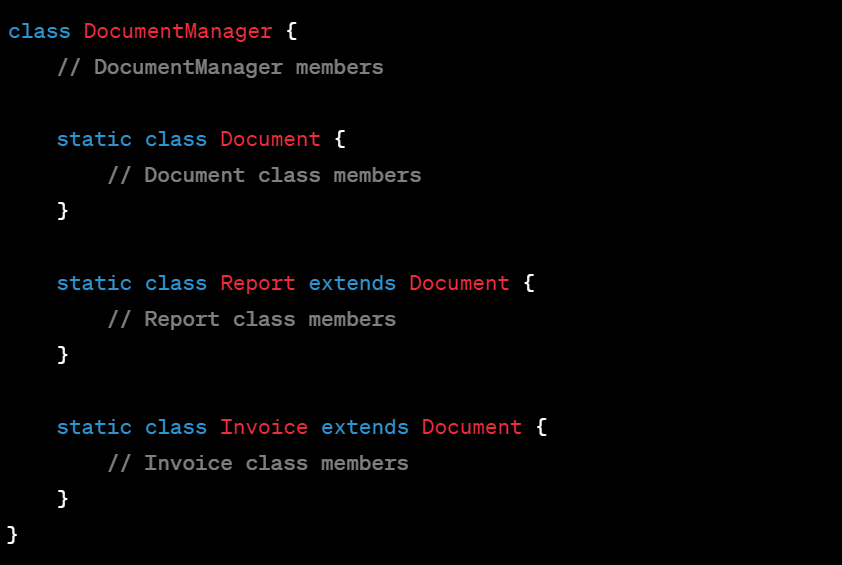
Considerations and Best Practices
- Limited Approach to Outer Group Members: Remember that constant nested classes have limited reach to the elements of the outer category. They are not able to approach the instance factors or procedures directly, as they lack any connection with a specific instance.
- Choosing the Right Level of Encapsulation: While they provide encapsulation, ensure that you strike the right balance between encapsulation and code readability. If a nested class’s functionality could be useful in other contexts, consider extracting it into a top-level category or package.
- Avoiding Overcomplexity: Be cautious not to create too many levels of nesting, as it can lead to code that is hard to navigate and understand. Reserve static nested classes for cases where encapsulation and arrangement truly make sense.
The Static Keyword Appliances
The “static” keyword in Java isn’t just a mere technicality; it’s a powerful tool that can be applied in various scenarios to enhance the structuring of the script, performance, and maintainability. Let’s take a look at some real-world use cases for this appliance, highlighting how it can be employed effectively to enhance your Java programming skills.
Singleton Pattern: Ensuring a Single Instance
The Singleton design pattern guarantees the presence of a sole example for a given group and establishes a universal access point to that example. This pattern proves exceptionally valuable when the goal is to regulate the creation of a resource-intensive entity, such as a database connection or a configuration manager. By employing the static keyword, the creation of a static method within the class becomes possible. This method subsequently furnishes the singular model while concurrently averting the generation of various samples.
Utility Classes: Providing Helper Functions
Static methods in utility categories offer a way to encapsulate commonly employed operations without the need to create instances of the category. These helper groups can provide a clean and organized way to group related functions together.
Constants: Defining Immutable Values
Fixed parameters and quantities, often referred to as constants, are useful for defining values that should remain unchanged throughout the execution of the program. By using the “static final” combination, you ensure that the value is shared among all samples of the class and cannot be modified.
Counters and Tracking: Monitoring Instances
Fixed variables can be employed to track the number of examples that have been created. This is particularly useful for debugging, performance monitoring, or managing resource allocation.
Factory Approaches: Abstracting Object Creation
Factory practices are the strategies used to generate samples of a class, abstracting the formation process. They can be helpful in scenarios where the exact category to instantiate is determined dynamically.
Summing Up
In conclusion, the “static” keyword in Java provides a powerful tool for creating class-level components that are accessible across the entirety samples of a class. By understanding its various use cases and best practices, developers can leverage static effectively to write efficient, organized, and maintainable code. Whether it’s sharing data, providing utility methods, or encapsulating related classes, the concept of static plays a crucial role in the world of Java programming.