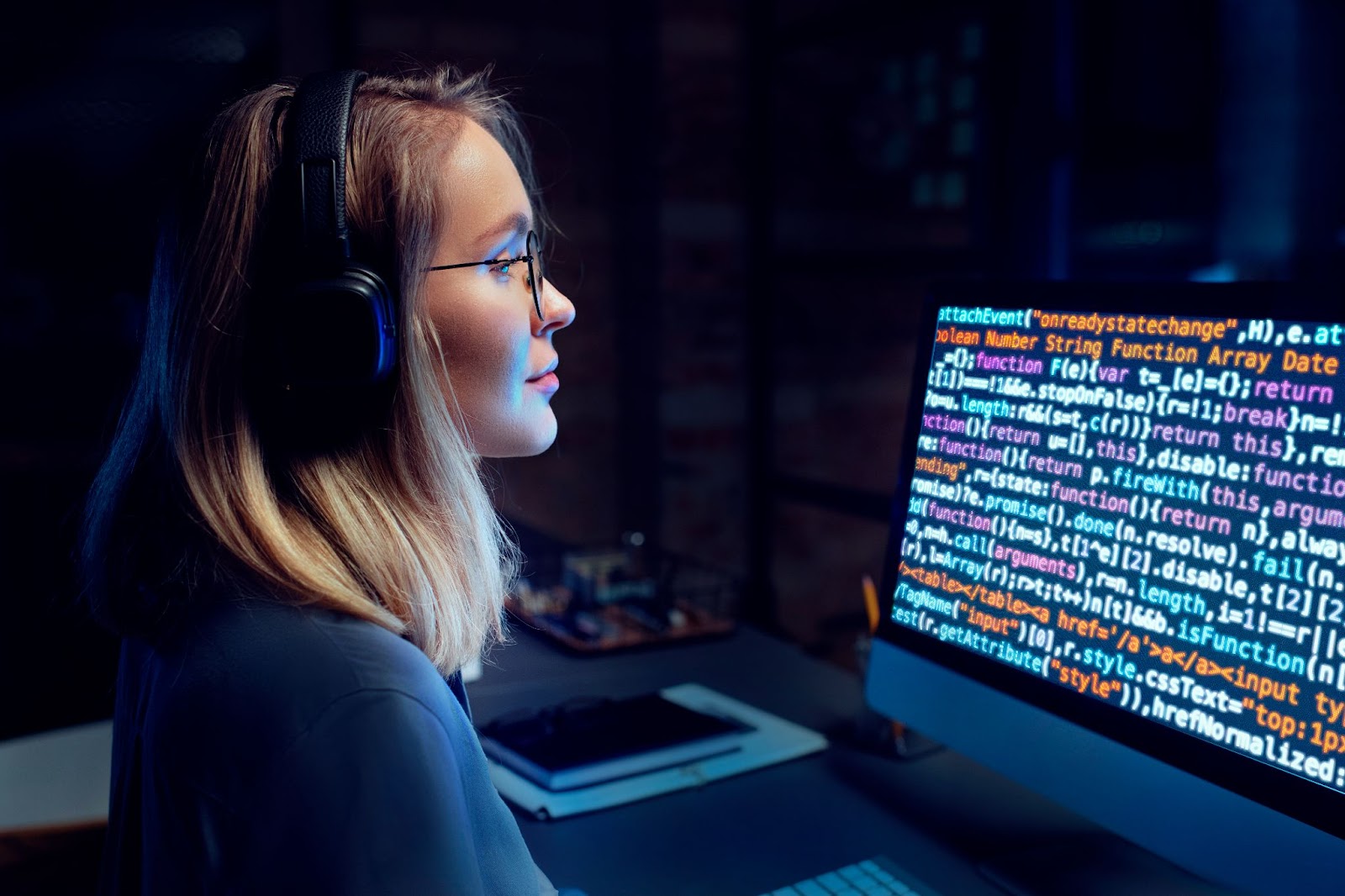
Transforming JSON Data into Java Objects
In the realm of modern software development, the efficient and seamless exchange of data between different programming languages and systems has become a cornerstone of success. JSON (JavaScript Object Notation) stands as one of the most prevalent data interchange formats, celebrated for its simplicity, human-readability, and widespread support across a multitude of platforms. On the other hand, Java, a stalwart of the programming world, boasts its own set of strengths, particularly in the realm of object-oriented programming and robust application development.
Whether you’re a seasoned developer looking to expand your skill set or a newcomer navigating the dynamic landscape of programming, this exploration into converting JSON to Java objects will provide valuable insights and hands-on guidance. By demystifying this crucial data transformation process, you’ll not only gain a deeper understanding of the inner workings of these technologies but also empower yourself to create more versatile, interoperable, and efficient software solutions.
Transforming JSON Data into Java Objects Using Jackson
When it comes to the seamless conversion of JSON data to Java objects, the Jackson library proves to be an indispensable tool. In this comprehensive guide, we’ll delve into the process of converting JSON to Java objects, starting with a simple Plain Old Java Object (POJO), and subsequently explore how to extend this technique to handling collections of objects. By the end, you’ll have a firm grasp of how to leverage the power of Jackson for efficient data conversion in your Java projects.
Adding the Jackson Dependency
Before we dive into the conversion process, let’s ensure we have the necessary dependencies in place. By adding the following Jackson dependencies to your project, you lay the foundation for seamless JSON-to-Java object conversion.
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.7.4</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.7.4</version>
</dependency>
Converting JSON to a Simple POJO
Let’s start by considering an illustrative example. Imagine we have the following JSON data:
{
“id”: “60”,
“codeId”: “1”,
“codeName”: “IT”,
“deleteFlag”: 0,
“sort”: “1”
}
Our goal is to convert this JSON structure into a corresponding Java object, which we’ll refer to as the MstCode object. This object is defined with fields to mirror the JSON attributes:
public class MstCode {
private int id;
private int codeId;
private String codeName;
private int deleteFlag;
private int sort;
// Getter and setter methods…
}
To achieve this conversion, we employ the ObjectMapper class from the Jackson library. This class provides a simple and efficient way to map JSON data to Java objects. Here’s how the process unfolds:
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.IOException;
public class JsonConverter {
public static void main(String[] args) {
String json = “{\”deleteFlag\”:0,\”codeId\”:\”1\”,\”codeName\”:\”IT\”,\”sort\”:\”1\”,\”id\”:\”60\”}”;
ObjectMapper mapper = new ObjectMapper();
try {
MstCode mstCode = mapper.readValue(json, MstCode.class);
System.out.print(mstCode.getCodeName());
} catch (IOException e) {
e.printStackTrace();
}
}
}
Upon executing this code snippet, you’ll witness the transformation of the JSON data into a Java object, facilitating the extraction of relevant attributes for further manipulation.
Converting JSON to a List of Objects
As we master the art of converting JSON to a single Java object, let’s expand our horizons to encompass scenarios where the JSON data encapsulates an array of objects. Consider the following JSON array:
[
{
“deleteFlag”: 0,
“codeId”: “1”,
“codeName”: “IT”,
“sort”: “1”,
“id”: “60”
},
{
“deleteFlag”: 0,
“codeId”: “2”,
“codeName”: “Accounting”,
“sort”: “2”,
“id”: “61”
},
{
“deleteFlag”: 0,
“codeId”: “3”,
“codeName”: “Support”,
“sort”: “3”,
“id”: “62”
}
]
Here, we have an array of JSON objects, each analogous to the MstCode object structure introduced earlier. Our objective is to harness the power of Jackson to convert this JSON array into a Java List of MstCode objects.
Expanding upon our JsonConverter class, we adapt the conversion process to accommodate collections:
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.IOException;
import java.util.List;
public class JsonConverter {
public static void main(String[] args) {
String json = “[{\”deleteFlag\”:0,\”codeId\”:\”1\”,\”codeName\”:\”IT\”,\”sort\”:\”1\”,\”id\”:\”60\”},{\”deleteFlag\”:0,\”codeId\”:\”2\”,\”codeName\”:\”Accounting\”,\”sort\”:\”2\”,\”id\”:\”61\”},{\”deleteFlag\”:0,\”codeId\”:\”3\”,\”codeName\”:\”Support\”,\”sort\”:\”3\”,\”id\”:\”62\”}]”;
ObjectMapper mapper = new ObjectMapper();
try {
List<MstCode> mstCodes = mapper.readValue(json, mapper.getTypeFactory().constructCollectionType(List.class, MstCode.class));
System.out.println(“Number of codes: ” + mstCodes.size());
System.out.println(“First code name: ” + mstCodes.get(0).getCodeName());
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this extended example, the focal point is the constructCollectionType method provided by the ObjectMapper class. By leveraging this method, we specify that the JSON array should be converted into a List of MstCode objects, thereby facilitating the retrieval and utilization of data from the array elements.
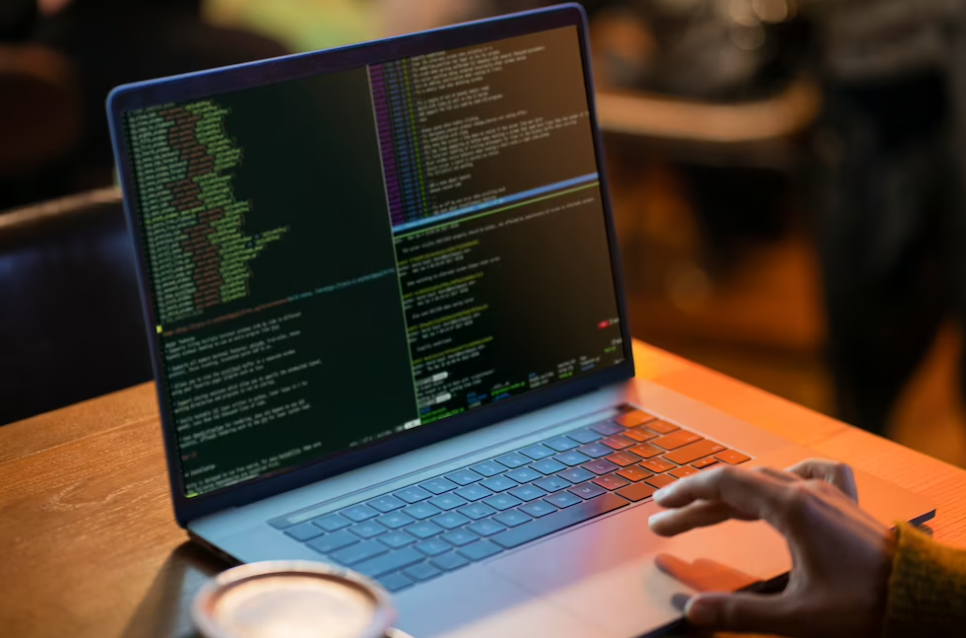
By effectively combining the insights gained from the conversion of both individual JSON objects and arrays of objects, you empower yourself to seamlessly integrate JSON data into your Java applications. The Jackson library’s ObjectMapper class is your steadfast companion on this journey, simplifying the intricacies of data conversion and enabling you to harness the potential of JSON data in your Java projects.
Conclusion
In conclusion, the ability to convert JSON (JavaScript Object Notation) into Java objects has proven to be a crucial aspect of modern software development. This process facilitates seamless communication and data interchange between different systems, enabling developers to efficiently work with data retrieved from APIs, databases, or other sources. By harnessing libraries and frameworks such as Jackson, Gson, or org.json, developers can effortlessly transform JSON data into Java objects, making it easier to manipulate, analyze, and utilize within their applications.
The conversion process not only saves valuable development time but also enhances code readability and maintainability. It allows developers to work with structured data in a familiar object-oriented manner, while also taking advantage of the dynamic nature of JSON to handle various data formats and structures. This capability empowers developers to build robust and flexible applications that can adapt to changing data requirements and evolve alongside the ever-evolving technology landscape.